mirror of
https://github.com/fluencelabs/js-libp2p-tcp
synced 2025-04-25 10:02:21 +00:00
Merge pull request #10 from noffle/master
Adds README, coverage, clean up, CircleCI.
This commit is contained in:
commit
ce6edb05a2
84
README.md
84
README.md
@ -11,6 +11,86 @@ js-libp2p-tcp
|
||||
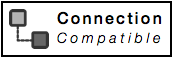
|
||||

|
||||
|
||||
> Node.js implementation of the TCP module that libp2p uses, which implements the [interface-connection](https://github.com/diasdavid/interface-connection) interface for dial/listen.
|
||||
> Node.js implementation of the TCP module that libp2p uses, which implements
|
||||
> the [interface-connection](https://github.com/diasdavid/interface-connection)
|
||||
> interface for dial/listen.
|
||||
|
||||
note: libp2p-tcp in Node.js is a very thin shim that adds the support to dial to a `multiaddr`. This small shim will enable libp2p to use other different transports.
|
||||
## Description
|
||||
|
||||
`libp2p-tcp` in Node.js is a very thin shim that adds the support to dial to a
|
||||
`multiaddr`. This small shim will enable libp2p to use other different
|
||||
transports.
|
||||
|
||||
## Example
|
||||
|
||||
```js
|
||||
const Tcp = require('libp2p-tcp')
|
||||
const multiaddr = require('multiaddr')
|
||||
|
||||
const mh1 = multiaddr('/ip4/127.0.0.1/tcp/9090')
|
||||
const mh2 = multiaddr('/ip6/::/tcp/9092')
|
||||
|
||||
const tcp = new Tcp()
|
||||
|
||||
tcp.createListener([mh1, mh2], function handler (socket) {
|
||||
console.log('connection')
|
||||
socket.end('bye')
|
||||
}, function ready () {
|
||||
console.log('ready')
|
||||
|
||||
const client = tcp.dial(mh1)
|
||||
client.pipe(process.stdout)
|
||||
client.on('end', () => {
|
||||
tcp.close(function(){})
|
||||
})
|
||||
})
|
||||
|
||||
```
|
||||
|
||||
outputs
|
||||
|
||||
```
|
||||
ready
|
||||
connection
|
||||
bye
|
||||
```
|
||||
|
||||
## Installation
|
||||
|
||||
### npm
|
||||
|
||||
```sh
|
||||
> npm i libp2p-tcp
|
||||
```
|
||||
|
||||
## API
|
||||
|
||||
```js
|
||||
const Tcp = require('libp2p-tcp')
|
||||
```
|
||||
|
||||
### var tcp = new Tcp()
|
||||
|
||||
Creates a new TCP object. This does nothing on its own but provide access to
|
||||
`dial` and `createListener`.
|
||||
|
||||
### tcp.createListener(multiaddrs, handler, callback)
|
||||
|
||||
Creates TCP servers that listen on the addresses described in the array
|
||||
`multiaddrs`. Each connection will call `handler` with a connection stream.
|
||||
`callback` is called once all servers are listening.
|
||||
|
||||
### tcp.dial(multiaddr, options={})
|
||||
|
||||
Connects to the multiaddress `multiaddr` using TCP, returning the socket stream.
|
||||
If `options.ready` is set to a function, it is called when a connection is
|
||||
established.
|
||||
|
||||
### tcp.close(callback)
|
||||
|
||||
Closes all the listening TCP servers, calling `callback` once all of them have
|
||||
been shut down.
|
||||
|
||||
## License
|
||||
|
||||
MIT
|
||||
|
8
circle.yml
Normal file
8
circle.yml
Normal file
@ -0,0 +1,8 @@
|
||||
dependencies:
|
||||
pre:
|
||||
# setup ipv6
|
||||
- sudo sysctl -w net.ipv6.conf.lo.disable_ipv6=0 net.ipv6.conf.default.disable_ipv6=0 net.ipv6.conf.all.disable_ipv6=0
|
||||
|
||||
machine:
|
||||
node:
|
||||
version: stable
|
10
src/index.js
10
src/index.js
@ -26,13 +26,7 @@ function TCP () {
|
||||
return conn
|
||||
}
|
||||
|
||||
this.createListener = (multiaddrs, options, handler, callback) => {
|
||||
if (typeof options === 'function') {
|
||||
callback = handler
|
||||
handler = options
|
||||
options = {}
|
||||
}
|
||||
|
||||
this.createListener = (multiaddrs, handler, callback) => {
|
||||
if (!Array.isArray(multiaddrs)) {
|
||||
multiaddrs = [multiaddrs]
|
||||
}
|
||||
@ -74,7 +68,7 @@ function TCP () {
|
||||
var count = 0
|
||||
listeners.forEach((listener) => {
|
||||
listener.close(() => {
|
||||
if (++count === listeners.length) {
|
||||
if (++count === listeners.length && callback) {
|
||||
callback()
|
||||
}
|
||||
})
|
||||
|
@ -15,6 +15,19 @@ describe('libp2p-tcp', function () {
|
||||
done()
|
||||
})
|
||||
|
||||
it('create without new', (done) => {
|
||||
tcp = TCPlibp2p()
|
||||
expect(tcp).to.exist
|
||||
done()
|
||||
})
|
||||
|
||||
it('close /wo listeners', (done) => {
|
||||
tcp = new TCPlibp2p()
|
||||
expect(tcp).to.exist
|
||||
expect(function () { tcp.close() }).to.throw(Error)
|
||||
done()
|
||||
})
|
||||
|
||||
it('listen', (done) => {
|
||||
const mh = multiaddr('/ip4/127.0.0.1/tcp/9090')
|
||||
tcp.createListener(mh, (socket) => {
|
||||
@ -46,13 +59,32 @@ describe('libp2p-tcp', function () {
|
||||
it('listen on several', (done) => {
|
||||
const mh1 = multiaddr('/ip4/127.0.0.1/tcp/9090')
|
||||
const mh2 = multiaddr('/ip4/127.0.0.1/tcp/9091')
|
||||
const mh3 = multiaddr('/ip6/::/tcp/9092')
|
||||
const tcp = new TCPlibp2p()
|
||||
|
||||
tcp.createListener([mh1, mh2], (socket) => {}, () => {
|
||||
tcp.createListener([mh1, mh2, mh3], (socket) => {}, () => {
|
||||
tcp.close(done)
|
||||
})
|
||||
})
|
||||
|
||||
it('dial ipv6', (done) => {
|
||||
const mh = multiaddr('/ip6/::/tcp/9091')
|
||||
var dialerObsAddrs
|
||||
|
||||
tcp.createListener(mh, (conn) => {
|
||||
expect(conn).to.exist
|
||||
dialerObsAddrs = conn.getObservedAddrs()
|
||||
conn.end()
|
||||
}, () => {
|
||||
const conn = tcp.dial(mh)
|
||||
conn.on('end', () => {
|
||||
expect(dialerObsAddrs.length).to.equal(1)
|
||||
tcp.close()
|
||||
done()
|
||||
})
|
||||
})
|
||||
})
|
||||
|
||||
it('get observed addrs', (done) => {
|
||||
const mh = multiaddr('/ip4/127.0.0.1/tcp/9090')
|
||||
var dialerObsAddrs
|
||||
@ -88,5 +120,12 @@ describe('libp2p-tcp', function () {
|
||||
done()
|
||||
})
|
||||
|
||||
it.skip('listen on IPv6', (done) => {})
|
||||
it('filter a valid addr for this transport', (done) => {
|
||||
const mh1 = multiaddr('/ip4/127.0.0.1/tcp/9090')
|
||||
|
||||
const valid = tcp.filter(mh1)
|
||||
expect(valid.length).to.equal(1)
|
||||
expect(valid[0]).to.deep.equal(mh1)
|
||||
done()
|
||||
})
|
||||
})
|
||||
|
Loading…
x
Reference in New Issue
Block a user